In the illustration below, i have created a docker image of jenkins with docker installed.
Here is the Dockerfile:
FROM jenkins/jenkins:lts
USER root
RUN apt-get update && \
apt-get -y install apt-transport-https \
ca-certificates \
curl \
gnupg2 \
software-properties-common && \
curl -fsSL https://download.docker.com/linux/$(. /etc/os-release;
echo "$ID")/gpg > /tmp/dkey; apt-key add /tmp/dkey && \
add-apt-repository \
"deb [arch=amd64] https://download.docker.com/linux/$(. /etc/os-release; echo "$ID") \
$(lsb_release -cs) \
stable" && \
apt-get update && \
apt-get -y install docker-ce
RUN apt-get install -y docker-ce
RUN usermod -a -G docker jenkins
USER jenkins
This Dockerfile is built from jenkins official image, install docker and give access to user jenkins build dockers.
Note: You can build this image, but is already in dockerhub gustavoapolinario/jenkins-docker.
Running jenkins with docker from host:
To run the container, you need to add a volume in docker run command.
… -v /var/run/docker.sock:/var/run/docker.sock …
This way the docker socket (used in your machine) wil be shared with the container.
The complete run command:
docker run --name jenkins-docker -p 8080:8080 -v /var/run/docker.sock:/var/run/docker.sock gustavoapolinario/jenkins-docker
After initializing the jenkins, complete the jenkins startup wizard and install the locale and blueocean plugins: Quick start with jenkins in docker.
Test the docker command by creating a Job:
Go to Jenkins homepage, click on “New Item”, select “Pipeline” and enter the job name as “docker-test”.
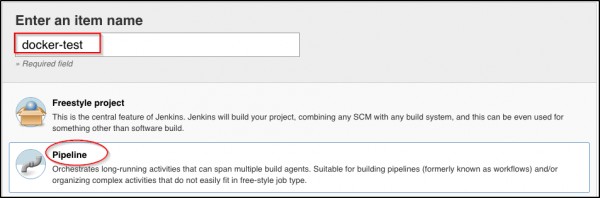
New pipeline Job.
Add this script inside the job:
pipeline { environment {
registry = "docker_hub_account/repository_name"
registryCredential = 'dockerhub'
} agent any stages {
stage('Building image') {
steps{
script {
docker.build registry + ":$BUILD_NUMBER"
}
}
}
}
}
The screen will be like this:
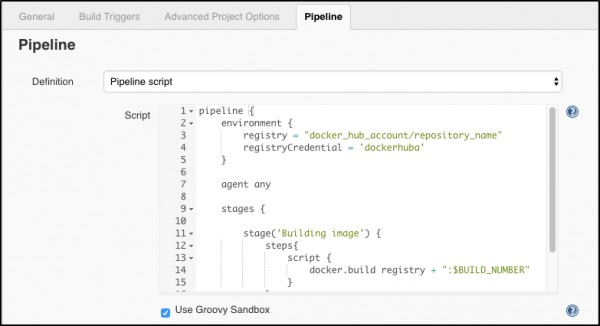
Pipeline in job config
Save the job.
Pipeline explanation:
In this pipeline, We have 2 environment variables to change the registry and the credential easily.
environment {
registry = "docker_hub_account/repository_name"
registryCredential = 'dockerhub'
}
The job will have one step.Docker build command will be run by utilizing the jenkins build number in the docker tag.
stages {
stage('Building image') {
steps{
script {
docker.build registry + ":$BUILD_NUMBER"
}
}
}
}
Test the docker command in the job:
Click on “Build Now” in job’s menu.
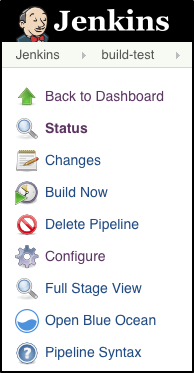
Job Menu
The job will fail.
Now click on build number (#1) -> “Console Output”.
You would see something like this:
Started by user GUSTAVO WILLY APOLINARIO DOMINGUES
Running in Durability level: MAX_SURVIVABILITY
[Pipeline] node
Running on Jenkins in /var/jenkins_home/workspace/docker-test
[Pipeline] {
[Pipeline] withEnv
[Pipeline] {
[Pipeline] stage
[Pipeline] { (Building image)
[Pipeline] script
[Pipeline] {
[Pipeline] sh
[teste234] Running shell script
+ docker build -t docker_hub_account/repository_name:1 .
unable to prepare context: unable to evaluate symlinks in Dockerfile path: lstat /var/jenkins_home/workspace/docker-test/Dockerfile: no such file or directory
[Pipeline] }
[Pipeline] // script
[Pipeline] }
[Pipeline] // stage
[Pipeline] }
[Pipeline] // withEnv
[Pipeline] }
[Pipeline] // node
[Pipeline] End of Pipeline
ERROR: script returned exit code 1
Finished: FAILURE
The error happens because the Dockerfile is not found. This will be resolved soon.
The docker command is executed and the jenkins has been successful in finding the command.
Creating a dockerhub repository:
Create a dockerhub account, if you don’t have yet.
Logged in dockerhub, click on “Create” > “Create Repository”.

Dockerhub menu to Create Repository
Put a name for your repository. For this example, use “docker-test”.
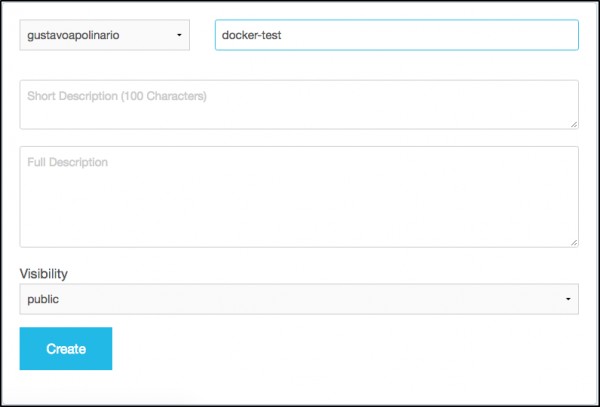
Creating Dockerhub Repository
After docker repository created, get the name to use in our pipeline. In this case, the name is “gustavoapolinario/docker-test”.
Create the dockerhub credential:
From jenkins home, click on “credentials” and “(global)”.
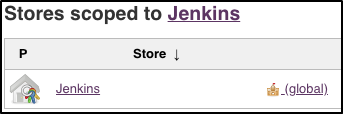
Credentials
Click on “Add Credentials” in left menu.

Put your credential and save it.
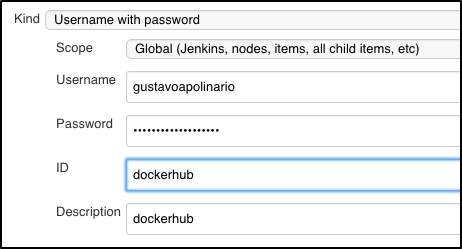
Remember to change the credential environment (registryCredential) if you didn’t put “dockerhub” in Credential ID.
The credential is configured.
Configuring the environment of dockerhub:
Alter the job pipeline. Go to jenkins home, click on job name (docker-test), click on “Configure” in job menu.
You need to change the following code:
environment {
registry = "docker_hub_account/repository_name"
registryCredential = ‘dockerhub’
}
Change the environment variable “registry” to your repository name. In this case it is “gustavoapolinario/docker-test”.
Change the environment variable “registryCredential” if necessary.
My environment is:
environment {
registry = "gustavoapolinario/docker-test"
registryCredential = ‘dockerhub’
}
Building the first docker image:
With dockerhub credential and repository created, the jenkins can send the docker image build to dockerhub (our docker repository).
In this example, let’s build a node.js application. We need a Dockerfile to the build.
Create a new step in pipeline to clone a git repository that has a Dockerfile inside.
stage('Cloning Git') {
steps {
git 'https://github.com/gustavoapolinario/microservices-node-example-todo-frontend.git'
}
}
The pipeline must be (but using your environment):
pipeline {
environment {
registry = "gustavoapolinario/docker-test"
registryCredential = ‘dockerhub’
}
agent any
stages {
stage('Cloning Git') {
steps {
git 'https://github.com/gustavoapolinario/microservices-node-example-todo-frontend.git'
}
}
stage('Building image') {
steps{
script {
docker.build registry + ":$BUILD_NUMBER"
}
}
}
}
}
Save and run it clicking on “Build Now”. The Stage view in jenkins job will change to this:
Deploying the docker image to dockerhub:
At this moment, we clone a git and build a docker image.
We need put this image in docker registry to pull it in other machines.
First, create a environment to save docker image informations.
dockerImage = ''
Change the build stage to save build information in environment.
dockerImage = docker.build registry + ":$BUILD_NUMBER"
Ceate a new stage to push the docker image builded to dockerhub.
stage('Deploy Image') {
steps{ script {
docker.withRegistry( '', registryCredential ) {
dockerImage.push()
}
}
}
}
After build and deploy, delete the image to cleanup your server space.
stage('Remove Unused docker image') {
steps{
sh "docker rmi $registry:$BUILD_NUMBER"
}
}
The final code will be (remember, using your environment):
pipeline {
environment {
registry = "gustavoapolinario/docker-test"
registryCredential = 'dockerhub'
dockerImage = ''
}
agent any
stages {
stage('Cloning Git') {
steps {
git 'https://github.com/gustavoapolinario/microservices-node-example-todo-frontend.git'
}
}
stage('Building image') {
steps{
script {
dockerImage = docker.build registry + ":$BUILD_NUMBER"
}
}
}
stage('Deploy Image') {
steps{
script {
docker.withRegistry( '', registryCredential ) {
dockerImage.push()
}
}
}
}
stage('Remove Unused docker image') {
steps{
sh "docker rmi $registry:$BUILD_NUMBER"
}
}
}
}
Save and run it.
The build is now complete and the image will be send to docker registry.
Ready to level up your Docker skills? Enroll now in our comprehensive Docker Course!