Hello @Sign,
It is simple to create contact form to send email or any other information and storing in mysql database.
Using INSERT INTO statement you can insert new data into a database table.
See the example below:
Output:
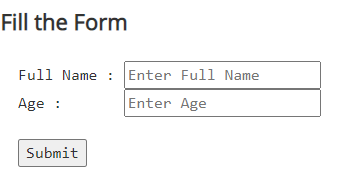

Step 1: Connection with Database
This is dbConn.php file which is used to connect the database.
This dbConn.php file will use everywhere, where you want to perform a task with the database.
Here it is connected with the local database and check database connected or not.
dbConn.php
<?php
$db = mysqli_connect("localhost","root","","testDB");
if(!$db)
{
die("Connection failed: " . mysqli_connect_error());
}
?>
Step 2: Creating HTML form and inserting data
Here's a simple HTML form which is index.php file. It has two text input and a submit button.
The INSERT INTO statement is used to add new data into the database. This insert query will execute passing through to the PHP mysqli_query() function to insert new data.
And also created a simple HTML form which has two input text and a submit button which is used to take data from users.
In the index.php file the dbConn.php file includes for connecting with the database and insert new data. The form has two field fullname and age which are get data from users and store into the database.
In this example the PHP code and the HTML form coded in the single page. The PHP script embedded with the HTML page.
index.php
<!DOCTYPE html>
<html>
<head>
<title>Add Records in Database</title>
</head>
<body>
<?php
include "dbConn.php"; // Using database connection file here
if(isset($_POST['submit']))
{
$fullname = $_POST['fullname'];
$age = $_POST['age'];
$insert = mysqli_query($db,"INSERT INTO `tblemp`(`fullname`, `age`) VALUES ('$fullname','$age')");
if(!$insert)
{
echo mysqli_error();
}
else
{
echo "Records added successfully.";
}
}
mysqli_close($db); // Close connection
?>
<h3>Fill the Form</h3>
<form method="POST">
Full Name : <input type="text" name="fullname" placeholder="Enter Full Name" Required>
<br/>
Age : <input type="text" name="age" placeholder="Enter Age" Required>
<br/>
<input type="submit" name="submit" value="Submit">
</form>
</body>
</html>
Write PHP code as a separate file
Step 1: Creating HTML Form
First of all creating HTML form which is index.php file. This form has two text boxes and a submit button for inserting data.
This form also has an action attribute which contains insert.php. This will perform when clicking on submit button.
index.php
<!DOCTYPE html>
<html>
<head>
<title>Add Records in Database</title>
</head>
<body>
<form action="insert.php" method="POST">
Full Name : <input type="text" name="fullname" placeholder="Enter Full Name" Required>
<br/>
Age : <input type="text" name="age" placeholder="Enter Age" Required>
<br/>
<input type="submit" name="submit" value="Submit">
</form>
</body>
</html>
Step 2: Connection with Database
This is dbConn.php file which connects the database.
dbConn.php
<?php
$db = mysqli_connect("localhost","root","","testDB");
if(!$db)
{
die("Connection failed: " . mysqli_connect_error());
}
?>
Step 3: Write PHP code for insert data
This is insert.php file. Here is written PHP code for inserting data when clicking on submit button.
insert.php
<?php
include "dbConn.php"; // Using database connection file here
if(isset($_POST['submit']))
{
$fullname = $_POST['fullname'];
$age = $_POST['age'];
$insert = mysqli_query($db,"INSERT INTO `tblemp`(`fullname`, `age`) VALUES ('$fullname','$age')");
if(!$insert)
{
echo mysqli_error();
}
else
{
echo "Records added successfully.";
}
}
mysqli_close($db); // Close connection
?>
Hope it helps!!
Thank you!