You can create a VPC using the following code:-
package com.ec2application.ec2application;
import com.amazonaws.auth.AWSCredentials;
import com.amazonaws.auth.AWSStaticCredentialsProvider;
import com.amazonaws.auth.BasicAWSCredentials;
import com.amazonaws.regions.Regions;
import com.amazonaws.services.ec2.AmazonEC2;
import com.amazonaws.services.ec2.AmazonEC2ClientBuilder;
import com.amazonaws.services.ec2.model.CreateVpcRequest;
import com.amazonaws.services.ec2.model.CreateVpcResult;
import com.amazonaws.services.ec2.model.Vpc;
public class CreateVPC
{
private static final AWSCredentials AWS_CREDENTIALS;
static {
// Your accesskey and secretkey
AWS_CREDENTIALS = new BasicAWSCredentials(
"Access Key",
"Security Key"
);
}
public static void main(String[] args) {
// Set up the amazon ec2 client
AmazonEC2 ec2Client = AmazonEC2ClientBuilder.standard()
.withCredentials(new AWSStaticCredentialsProvider(AWS_CREDENTIALS))
.withRegion(Regions.US_EAST_1)
.build();
System.out.println("Creating a VPC");
CreateVpcRequest newVPC = new CreateVpcRequest("In");
newVPC.setCidrBlock("10.0.0.0/16");
CreateVpcResult res = ec2Client.createVpc(newVPC);
Vpc vp = res.getVpc();
String vpcId = vp.getVpcId();
System.out.println("Created VPC " + vpcId);
}
}
This will generate an output like this:-
Creating a VPC
Created VPC vpc-0ba1336c724dc62cc
Now you can verify whether your VPC is created or not on AWS Console.
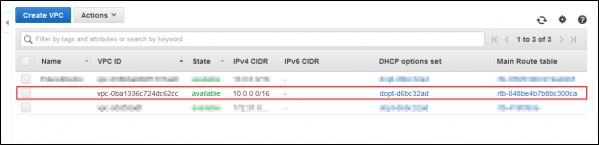
You can see that your VPC is created successfully.
Hope this helps!
Want to learn more about Java SDK? Enroll for the Java course online here!
Thanks!