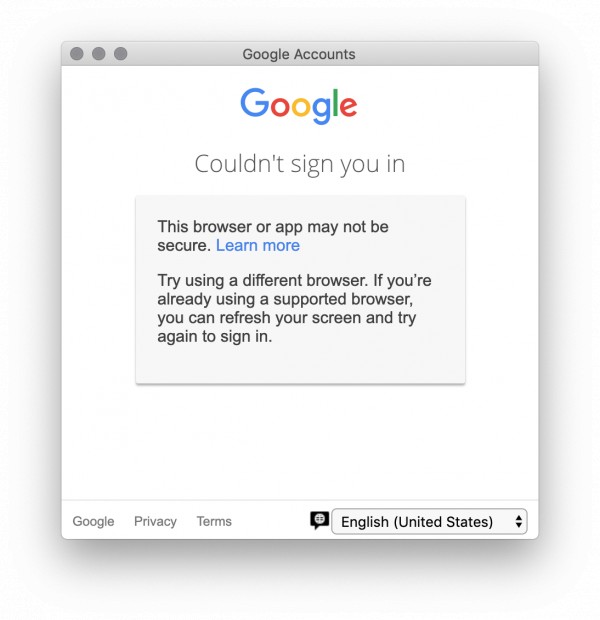
import org.openqa.selenium.By;
import org.openqa.selenium.JavascriptExecutor;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.WrapsElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.chrome.ChromeOptions;
import org.openqa.selenium.ie.InternetExplorerDriver;
import org.openqa.selenium.remote.DesiredCapabilities;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
import org.testng.annotations.Test;
import autoIT.AutoITClass;
public class sample1
{
public static void main(String[] args) throws Exception
{
DesiredCapabilities capabilities = DesiredCapabilities.chrome();
ChromeOptions options = new ChromeOptions();
options.addArguments("incognito");
capabilities.setCapability(ChromeOptions.CAPABILITY, options);
System.setProperty("webdriver.chrome.driver","D:\\Testing\\softwares\\chromedriver.exe");
WebDriver driver=new ChromeDriver(capabilities);
driver.get("https://firebaseapp.com");
driver.manage().timeouts().implicitlyWait(30,TimeUnit.SECONDS);
WebElement patient = driver.findElement(By.xpath("//span[contains(text(),'Sign in as Patient')]"));
patient.click();
try
{
driver.get("https://accounts.google.com/signin");
WebElement email = driver.findElement(By.xpath("//input[@type=\"email\"]"));
email.sendKeys("myemail@gmail.com");
WebDriverWait wait=new WebDriverWait(driver, 5);
WebElement emailNext = driver.findElement(By.xpath("//span[contains(text(),'Next')]"));
emailNext.click();
WebElement element = wait.until(ExpectedConditions.visibilityOfElementLocated(By.id("password")));
driver.findElement(By.xpath("//input[@name='password']")).sendKeys("your password");
driver.findElement(By.xpath("//span[@class='RveJvd snByac']")).click();
driver.manage().timeouts().implicitlyWait(30,TimeUnit.SECONDS);
} catch (Exception e) {
// TODO Auto-generated catch block
System.out.println(e.getMessage());
e.printStackTrace();
}
}
}