Explanation of compile and link process don't come with an example.Let's look at directive named sample which appears in the following markup.
<div ng-repeat="i in [0,1,2]">
<simple>
<div>Inner content</div>
</simple>
</div>
The directive appears once inside an ng-repeat and will need to render three times.
Now,what we want to focus on is how and when the various directive functions execute, as well as the arguments to the compile and linking functions.
To see what happens we’ll use the following shell of a directive definition.
app.directive("simple", function(){
return {
restrict: "EA",
transclude:true,
template:"<div>{{label}}<div ng-transclude></div></div>",
compile: function(element, attributes){
return {
pre: function(scope, element, attributes, controller, transcludeFn){
},
post: function(scope, element, attributes, controller, transcludeFn){
} } },
controller: function($scope){
}
};
});
Compile Executes Once
The first function to execute in the simple directive during view rendering will be the compile function.
The compile function will receive the simple element as a jqLite reference, and the element contents will
look like the content in the picture,
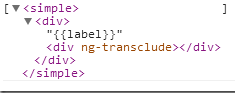
|
This compile function in the sample above returns an object with the pre and post linking functions.
However, many times we don’t need to hook into the compilation phase, so we can have a link function instead of a compile function.
app.directive("simple", function(){
return {
//...
link: function(scope, element, attributes){
},
controller: function($scope, $element){
}
};
});
A link function will behave like the post-link function described below.
Loop Three Times
Since the ngRepeat requires three copies of simple, we will now execute the following functions once for each instance.
Controller Function Executes
The first function to execute for each instance is the controller function.
It is here where the code can initialize a scope object as any good controller function will do.
Note the controller can also take an $element argument and receive a reference to the simple element clone that will appear in the DOM.
Pre-link Executes
By the time we reach the pre-link function (the function attached to the pre property of the object returned from compile),
we’ll have both a scope initialized by the controller function, and a reference to a real element that will appear in the DOM.
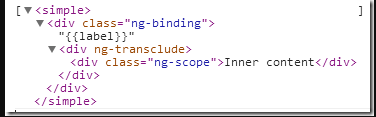
|
Post-link Executes
Post link is the last function to execute. Now the transclusion is complete, the template is linked to a scope, and the view
will update with data bound values after the next digest cycle .
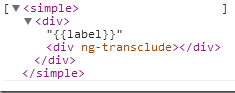
In post-link it is safe to manipulate the DOM, attach event handlers, inspect child elements, and
setup observations on attributes and watches on the scope.
To Sum Up: First Complie then controls move to controller,after that pre-linking
and then finally post-linking process takes place in Angularjs
Hope this helps!
To know more about Angular, It's recommended to join Angular JS course today.
Thanks.