Hey, to capture network network traffic of a specific page using Selenium Webdriver:
public class CaptureNetworkTraffic {
public static WebDriver driver;
public static String driverPath = "C:\\\\Users\\\\Abha_Rathour\\\\Downloads\\\\ExtractedFiles\\\\chromedriver_win32\\\\chromedriver.exe";
@SuppressWarnings("deprecation")
public static void main(String[] args) {
System.setProperty("webdriver.chrome.driver", driverPath);
DesiredCapabilities caps = DesiredCapabilities.chrome();
LoggingPreferences logPrefs = new LoggingPreferences();
logPrefs.enable(LogType.PERFORMANCE, Level.INFO);
caps.setCapability(CapabilityType.LOGGING_PREFS, logPrefs);
driver= new ChromeDriver(caps);
driver.get("https://www.edureka.co/");
List<LogEntry> entries = driver.manage().logs().get(LogType.PERFORMANCE).getAll();
System.out.println(entries.size() + " " + LogType.PERFORMANCE + " log entries found");
for (LogEntry entry : entries) {
System.out.println(new Date(entry.getTimestamp()) + " " + entry.getLevel() + " " + entry.getMessage());
}
driver.close();
driver.quit();
}
}
This will produce following output:
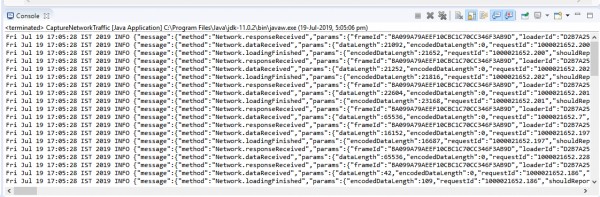
For further understanding, you can refer to the Selenium Course.